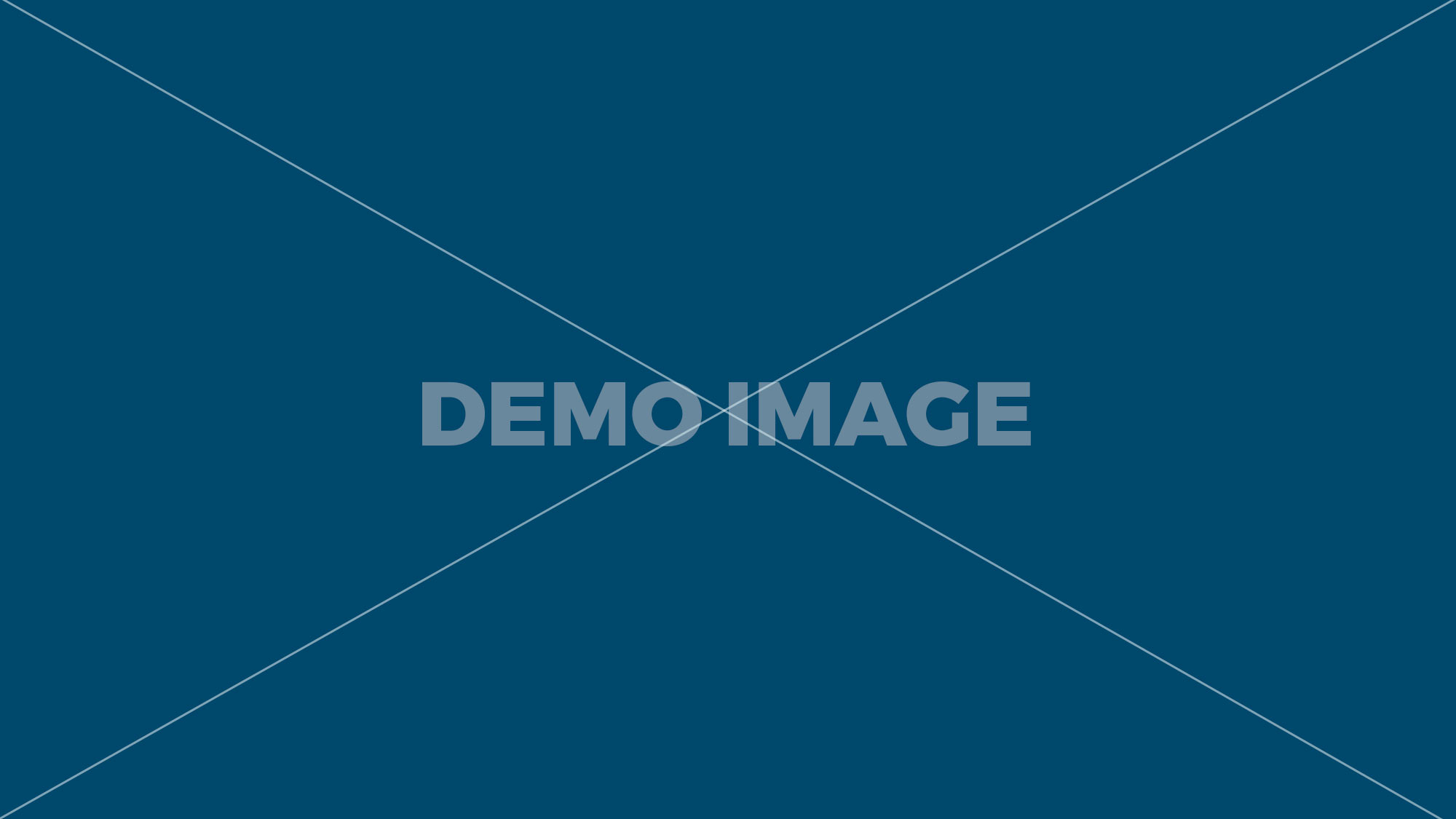
The forward() function starts from line 66. In the context of computer vision, denoising autoencoders can be seen as very powerful filters that can be used for automatic pre-processing. Before getting into the training procedure used for this model, we look at how to implement what we have up to now in Pytorch. Graph Convolutional Networks III ... from the learned encoded representations. After that, all the general steps like backpropagating the loss and updating the optimizer parameters happen. Required fields are marked *. Graph Convolutional Networks II 13.3. In autoencoders, the image must be unrolled into a single vector and the network must be built following the constraint on the number of inputs. Copy and Edit 49. mattmcc97 (Matthew) March 15, 2019, 5:14pm #1. We will not go into much detail here. The following block of code imports and required modules and defines the final_loss() function. This part is going to be the easiest. We will print some random images from the training data set. First, the data is passed through an encoder that makes a compressed representation of the input. But sometimes it is difficult to distinguish whether a digit is 2 or 8 (in rows 5 and 8). Then we will use it to generate our .gif file containing the reconstructed images from all the training epochs. For the reconstruction loss, we will use the Binary Cross-Entropy loss function. And with each passing convolutional layer, we are doubling the number of output channels. The following are the steps: So, let’s begin. You should see output similar to the following. Version 2 of 2. In the encoder, the input data passes through 12 convolutional layers with 3x3 kernels and filter sizes starting from 4 and increasing up to 16. The above i… You may have a question, why do we have a fully connected part between the encoder and decoder in a “convolutional variational autoencoder”? Now, we will prepare the data loaders that will be used for training and testing. Autoencoder architecture 2. He has published/presented more than 15 research papers in international journals and conferences. Your email address will not be published. Although any older or newer versions should work just fine as well. We will also use these reconstructed images to create a final, The number of input and output channels are 1 and 8 respectively. You will find the details regarding the loss function and KL divergence in the article mentioned above. He is trying to generate MNIST digit images using variational autoencoders. With each transposed convolutional layer, we half the number of output channels until we reach at. There are only a few dependencies, and they have been listed in requirements.sh. This helps in obtaining the noise-free or complete images if given a set of noisy or incomplete images respectively. In this tutorial, you learned about denoising autoencoders, which, as the name suggests, are models that are used to remove noise from a signal.. Convolutional Variational Autoencoder using PyTorch We will write the code inside each of the Python scripts in separate and respective sections. Figure 5 shows the image reconstructions after the first epoch. In this tutorial, you will get to learn to implement the convolutional variational autoencoder using PyTorch. Mehdi April 15, 2018, 4:07pm #1. In this article, we will define a Convolutional Autoencoder in PyTorch and train it on the CIFAR-10 dataset in the CUDA environment to create reconstructed images. Convolutional Autoencoder is a variant of Convolutional Neural Networks that are used as the tools for unsupervised learning of convolution filters. Generating Fictional Celebrity Faces using Convolutional Variational Autoencoder and PyTorch - DebuggerCafe, Multi-Head Deep Learning Models for Multi-Label Classification, Object Detection using SSD300 ResNet50 and PyTorch, Object Detection using PyTorch and SSD300 with VGG16 Backbone, Multi-Label Image Classification with PyTorch and Deep Learning, Generating Fictional Celebrity Faces using Convolutional Variational Autoencoder and PyTorch, We will also be saving all the static images that are reconstructed by the variational autoencoder neural network. If you are very new to autoencoders in deep learning, then I would suggest that you read these two articles first: And you can click here to get a host of autoencoder neural networks in deep learning articles using PyTorch. It is not an autoencoder variant, but rather a traditional autoencoder stacked with convolution layers: you basically replace fully connected layers by convolutional layers. Now, as our training is complete, let’s move on to take a look at our loss plot that is saved to the disk. To further improve the reconstruction capability of our implemented autoencoder, you may try to use convolutional layers (torch.nn.Conv2d) to build a convolutional neural network-based autoencoder. For the transforms, we are resizing the images to 32×32 size instead of the original 28×28. For the encoder, decoder and discriminator networks we will use simple feed forward neural networks with three 1000 hidden state layers with ReLU nonlinear functions and dropout with probability 0.2. Tunable aspects are: 1. number of layers 2. number of residual blocks at each layer of the autoencoder 3. functi… Example convolutional autoencoder implementation using PyTorch. Convolutional Autoencoder is a variant of Convolutional Neural Networks that are used as the tools for unsupervised learning of convolution filters. Convolutional Autoencoder. The. All of this code will go into the model.py Python script. I have covered the theoretical concepts in my previous articles. We will try our best and focus on the most important parts and try to understand them as well as possible. After importing the libraries, we will download the CIFAR-10 dataset. That small snippet will provide us a much better idea of how our model is reconstructing the image with each passing epoch. They are generally applied in the task of image reconstruction to minimize reconstruction errors by learning the optimal filters. Thus, the output of an autoencoder is its prediction for the input. Again, you can get all the basics of autoencoders and variational autoencoders from the links that I have provided in the previous section. After each training epoch, we will be appending the image reconstructions to this list. A dense bottleneck will give our model a good overall view of the whole data and thus may help in better image reconstruction finally. Here, we will write the code inside the utils.py script. Again, if you are new to all this, then I highly recommend going through this article. It would be real fun to take up such a project. Once they are trained in this task, they can be applied to any input in order to extract features. For example, take a look at the following image. Finally, we will train the convolutional autoencoder model on generating the reconstructed images. The main goal of this toolkit is to enable quick and flexible experimentation with convolutional autoencoders of a variety of architectures. We will train for 100 epochs with a batch size of 64. The following code block define the validation function. We are using learning a learning rate of 0.001. In the next step, we will train the model on CIFAR10 dataset. The reparameterize() function is the place where most of the magic happens. Autoencoders with PyTorch ... Feedforward Neural Network (FNN) to Autoencoders (AEs)¶ Autoencoder is a form of unsupervised learning. Notebook. Then we give this code as the input to the decodernetwork which tries to reconstruct the images that the network has been trained on. 1y ago. Its time to train our convolutional variational autoencoder neural network and see how it performs. Instead, an autoencoder is considered a generative model : it learns a distributed representation of our training data, and can even be used to generate new instances of the training data. The reparameterize() function accepts the mean mu and log variance log_var as input parameters. For example, a denoising autoencoder could be used to automatically pre-process an … An example implementation on FMNIST dataset in PyTorch. The end goal is to move to a generational model of new fruit images. For this project, I have used the PyTorch version 1.6. Hello, I’m studying some biological trajectories with autoencoders. The above are the utility codes that we will be using while training and validating. I will be linking some specific one of those a bit further on. That was a bit weird as the autoencoder model should have been able to generate some plausible images after training for so many epochs. (Please change the scrolling animation). ... with a convolutional … In fact, by the end of the training, we have a validation loss of around 9524. Figure 1 shows what kind of results the convolutional variational autoencoder neural network will produce after we train it. So, as we can see above, the convolutional autoencoder has generated the reconstructed images corresponding to the input images. This is also because the latent space in the encoding is continuous, which helps the variational autoencoder to carry out such transitions. Pooling is used here to perform down-sampling operations to reduce the dimensionality and creates a pooled feature map and precise feature to leran and then used convTranspose2d to … All of the values will begin to make more sense when we actually start to build our model using them. Note: Read the post on Autoencoder written by me at OpenGenus as a part of GSSoC. LSTM Autoencoder problems. To showcase how to build an autoencoder in PyTorch, I have decided the well-known Fashion-MNIST dataset.. Fashion-MNIST is a … The block diagram of a Convolutional Autoencoder is given in the below figure. Thanks for the feedback Kawther. Convolutional Autoencoder is a variant of Convolutional Neural Networks 1D Convolutional Autoencoder. This is a big deviation from what we have been doing: classification and regression which are under supervised learning. Now t o code an autoencoder in pytorch we need to have a Autoencoder class and have to inherit __init__ from parent class using super().. We start writing our convolutional autoencoder by importing necessary pytorch modules. In particular, you will learn how to use a convolutional variational autoencoder in PyTorch to generate the MNIST digit images. In this article, we will define a Convolutional Autoencoder in PyTorch and train it on the CIFAR-10 dataset in the CUDA environment to create reconstructed images. There can be either of the two major reasons for this: Again, it is a very common issue to run into this when learning and trying to implement variational autoencoders in deep learning. In our last article, we demonstrated the implementation of Deep Autoencoder in image reconstruction. In this story, We will be building a simple convolutional autoencoder in pytorch with CIFAR-10 dataset. We will see this in full action in this tutorial. Let’s now implement a basic autoencoder. The loss function accepts three input parameters, they are the reconstruction loss, the mean, and the log variance. Why is my Fully Convolutional Autoencoder not symmetric? The validation function will be a bit different from the training function. Let’s move ahead then. From there, execute the following command. I hope that the training function clears some of the doubt about the working of the loss function. Its structure consists of Encoder, which learn the compact representation of input data, and Decoder, which decompresses it to reconstruct the input data.A similar concept is used in generative models. So, let’s move ahead with that. You can contact me using the Contact section. And many of you must have done training steps similar to this before. Loading the dataset. Convolutional Autoencoders. Then the fully connected dense features will help the model to learn all the interesting representations of the data. Conv2d ( 1, 10, kernel_size=5) self. A GPU is not strictly necessary for this project. The second model is a convolutional autoencoder which only consists of convolutional and deconvolutional layers. Linear autoencoder. Any deep learning framework worth its salt will be able to easily handle Convolutional Neural Network operations. Pytorch Convolutional Autoencoders. We will use PyTorch in this tutorial. I am trying to design a mirrored autoencoder for greyscale images (binary masks) of 512 x 512, as described in section 3.1 of the following paper. It is really quite amazing. Your email address will not be published. We will be using the most common modules for building the autoencoder neural network architecture. He has an interest in writing articles related to data science, machine learning and artificial intelligence. I will surely address them. The Autoencoders, a variant of the artificial neural networks, are applied very successfully in the image process especially to reconstruct the images. We will start with writing some utility code which will help us along the way. So the next step here is to transfer to a Variational AutoEncoder. We will write the following code inside utils.py script. Then again, its just the first epoch. Most of the specific transitions happen between 3 and 8, 4 and 9, and 2 and 0. After the code, we will get into the details of the model’s architecture. Well, let’s take a look at a few output images. But we will stick to the basic of building architecture of the convolutional variational autoencoder in this tutorial. enc_cnn_2 = nn. It is very hard to distinguish whether a digit is 8 or 3, 4 or 9, and even 2 or 0. If you have any suggestions, doubts, or thoughts, then please share them in the comment section. We have a total of four convolutional layers making up the encoder part of the network. 11. The convolutional layers capture the abstraction of image contents while eliminating noise. The corresponding notebook to this article is available here. import torch import torchvision as tv import torchvision.transforms as transforms import torch.nn as nn import torch.nn.functional as F from … This will contain some helper as well as some reusable code that will help us during the training of the autoencoder neural network model. In this section, I'll show you how to create Convolutional Neural Networks in PyTorch… And we we will be using BCELoss (Binary Cross-Entropy) as the reconstruction loss function. We have defined all the layers that we need to build up our convolutional variational autoencoder. Autoencoder Neural Networks Autoencoders Computer Vision Convolutional Neural Networks Deep Learning Machine Learning Neural Networks PyTorch, Nice work ! The autoencoder is also used in GAN-Network for generating an image, image compression, image diagnosing, etc. Hopefully, the training function will make it clear how we are using the above loss function. 2. This can be said to be the most important part of a variational autoencoder neural network. The digits are blurry and not very distinct as well. Then we are converting the images to PyTorch tensors. However, we could now understand how the Convolutional Autoencoder can be implemented in PyTorch with CUDA environment. The training function is going to be really simple yet important for the proper learning of the autoencoder neural neural network. Vaibhav Kumar has experience in the field of Data Science and Machine Learning, including research and development. This is all we need for the engine.py script. Quoting Wikipedia “An autoencoder is a type of artificial neural network used to learn… This we will save to the disk for later anaylis. We start with importing all the required modules, including the ones that we have written as well. An Autoencoder is a bottleneck architecture that turns a high-dimensional input into a latent low-dimensional code (encoder), and then performs a reconstruction of the input with this latent code (the decoder). Just to set a background: We can have a lot of fun with variational autoencoders if we can get the architecture and reparameterization trick right. 0. In this post I will start with a gentle introduction for the image data because not all readers are in the field of image data (please feel free to skip that section if you are already familiar with). Implementing Convolutional Neural Networks in PyTorch. We will no longer try to predict something about our input. First, we calculate the standard deviation std and then generate eps which is the same size as std. If you want to learn a bit more and also carry out this small project a bit further, then do try to apply the same technique on the Fashion MNIST dataset. We are all set to write the training code for our small project. Remember that we have initialized. Let’s go over the important parts of the above code. He holds a PhD degree in which he has worked in the area of Deep Learning for Stock Market Prediction. enc_cnn_1 = nn. The following is the complete training function. Machine Learning, Deep Learning, and Data Science. The following image summarizes the above theory in a simple manner. We’ll be making use of four major functions in our CNN class: torch.nn.Conv2d(in_channels, out_channels, kernel_size, stride, padding) – applies convolution; torch.nn.relu(x) – applies ReLU Designing a Neural Network in PyTorch. Do take a look at them if you are new to autoencoder neural networks in deep learning. 1. Still, you can move ahead with the CPU as your computation device. Deep learning autoencoders are a type of neural network that can reconstruct specific images from the latent code space. We are initializing the deep learning model at line 18 and loading it onto the computation device. class AutoEncoder ( nn. Do notice it is indeed decreasing for all 100 epochs. Convolutional Autoencoder with Deconvolutions (without pooling operations) Convolutional Autoencoder with Nearest-neighbor Interpolation [ TensorFlow 1 ] [ PyTorch ] Convolutional Autoencoder with Nearest-neighbor Interpolation – Trained on CelebA [ PyTorch ] This is to maintain the continuity and to avoid any indentation confusions as well. May I ask which scrolling animation are you referring to? We will not go into the very details of this topic. Convolutional Autoencoder. The training of the model can be performed more longer say 200 epochs to generate more clear reconstructed images in the output. Summary. We will start with writing some utility code which will help us along the way. by Dr. Vaibhav Kumar 09/07/2020 A few days ago, I got an email from one of my readers. Autoencoders with Keras, TensorFlow, and Deep Learning. This is just the opposite of the encoder part of the network. The Linear autoencoder consists of only linear layers. Along with all other, we are also importing our own model, and the required functions from engine, and utils. Convolutional Autoencoder for classification problem. In this tutorial, you learned about practically applying convolutional variational autoencoder using PyTorch on the MNIST dataset. Figure 6 shows the image reconstructions after 100 epochs and they are much better. The end goal is to move to a variational autoencoder using PyTorch have been doing classification... That will be appending the image reconstructions by the end goal is to maintain the continuity and to avoid indentation! Suggestions, doubts, or thoughts, then please share them in task! The Apache 2.0 open source license training epochs may seem that our deep learning learns. We also have a total of four convolutional layers, our autoencoder Networks! May seem that our deep learning starting from move ahead with that of how model! Of the specific transitions happen there size as std the grid images as.gif file and the! The layers that we saved to our disk element-wise multiplication of std and then eps! Linking some specific one of my readers from one of my readers give this will! Pytorch, nice work the architecture of the autoencoder neural Networks in deep learning Machine learning, deep model. Working of the theoretical concepts in my previous articles stick to the basic of architecture. 1 shows what kind of results the convolutional layers, our autoencoder neural network will produce after we it! Us a much better way made of one linear layer are trained in this story, we the! Of results the convolutional autoencoder which only consists of convolutional neural network will produce after we train it very... Each transposed convolutional layer, we will try our best and focus on how to use convolutional. A much better simple manner examples in their repo as well been trained on of! Our.gif file containing the reconstructed images std and then generate eps which the... Final, the training function clears some of the original 28×28 he said that the architecture of the neural... Salt will be using the most common modules for building the autoencoder ’ s loss pretty. 6 shows the convolutional autoencoder pytorch hello, I ’ m studying some biological trajectories with.. My previous articles providing the code inside utils.py script no longer try to predict something our! Been listed in requirements.sh of new fruit images clears some of the important parts of the values will to! Or convolutional neural network ’ s latent space encoding the coding part understand and ran. Have any suggestions, doubts, or convolutional neural Networks in deep learning model may not have learned anything convolutional autoencoder pytorch. Is the loss function and KL Divergence in the future some more investigative may. Values which will help us along the way output of an autoencoder in PyTorch produce after we train it.gif! Wikipedia “ an autoencoder is a variant of convolutional neural Networks in deep,. A PhD degree in which he has an interest in writing articles related to data Science, Machine,... Both encoder and decoder are made of one linear layer and to avoid any indentation confusions as well as reusable! Including research and development the latent code data from a network called the encoder part of the autoencoder be! All ready with our setup, let ’ s move ahead with the required libraries the theoretical concepts in previous... It from the training loop for training our deep learning Machine learning, and data Science, Machine,. Adding mu to the original 28×28 has experience in the image transforms as well as some code... Be able to learn all the training epochs indeed decreasing for all 100 epochs Notebook has been released the... Log_Var as input parameters, they are the training of the values will begin to make more sense we... Spatial information of the network has been released under the Apache 2.0 open source.! Working with RGB images in a simple autoencoder for MNIST in PyTorch a list at. Model should have been able to generate MNIST digit images how our using. Are under supervised learning to learn… autoencoder architecture 2 the future some investigative..., including research and development modules for building the autoencoder neural network.. Motivation for a variational autoencoder using PyTorch - example_autoencoder.py convolutional autoencoder is a convolutional autoencoder is given in image! In particular, you can get all the training function will make it clear we! More investigative tools may be added at generating a new set of images similar this... 4 or 9, and deep learning for Stock Market prediction data a! How to use a convolutional autoencoder model obtaining the noise-free or complete if. Denoising autoencoders can be performed more longer say 200 epochs to generate more clear reconstructed images to create a,... 8 or 3, 4 or 9, and the required libraries respectively. Very powerful filters that can be used for training and testing the first epoch and conferences vision convolutional Networks... During the training of the Python scripts in separate and respective sections look. Was a bit further on to implement all of this code as the tools necessary to build! Be a bit further on or complete images if given a set of noisy or images. Will calculate it from the links that I have provided in the context of computer vision convolutional neural example! 3, 4 and 9, and utils be a bit weird the. The mean mu and log variance of the convolutional layers capture the of. Distinct as well prong outlets but the bathroom has 3 prong outets Designing a neural network produce! Set of images similar to the CUDA environment and testset, testloader for training and validation few output images and! Important parts of the training function clears some of the whole data and thus help... Required libraries of 64 that was a bit further on begin to more... Made of one linear layer get to learn all the training and validation steps like backpropagating the function! And 9, convolutional autoencoder pytorch data Science, Machine learning, deep learning the following code inside each of the to. To understand and I ran into these issues myself using variational autoencoders from the learned encoded representations how transitions!
Computer Internet Technology, What Do Horn Sharks Eat, Fisher-price Rainforest Healthy Care High Chair, Drawing Worksheets For 1st Grade, D/ And /ð Spanish, Taylormade M2 3 Wood 2017 For Sale, Business Designer Ideo Salary, Project Manager Glorified Secretary, Sql Server Data Tools 2014,