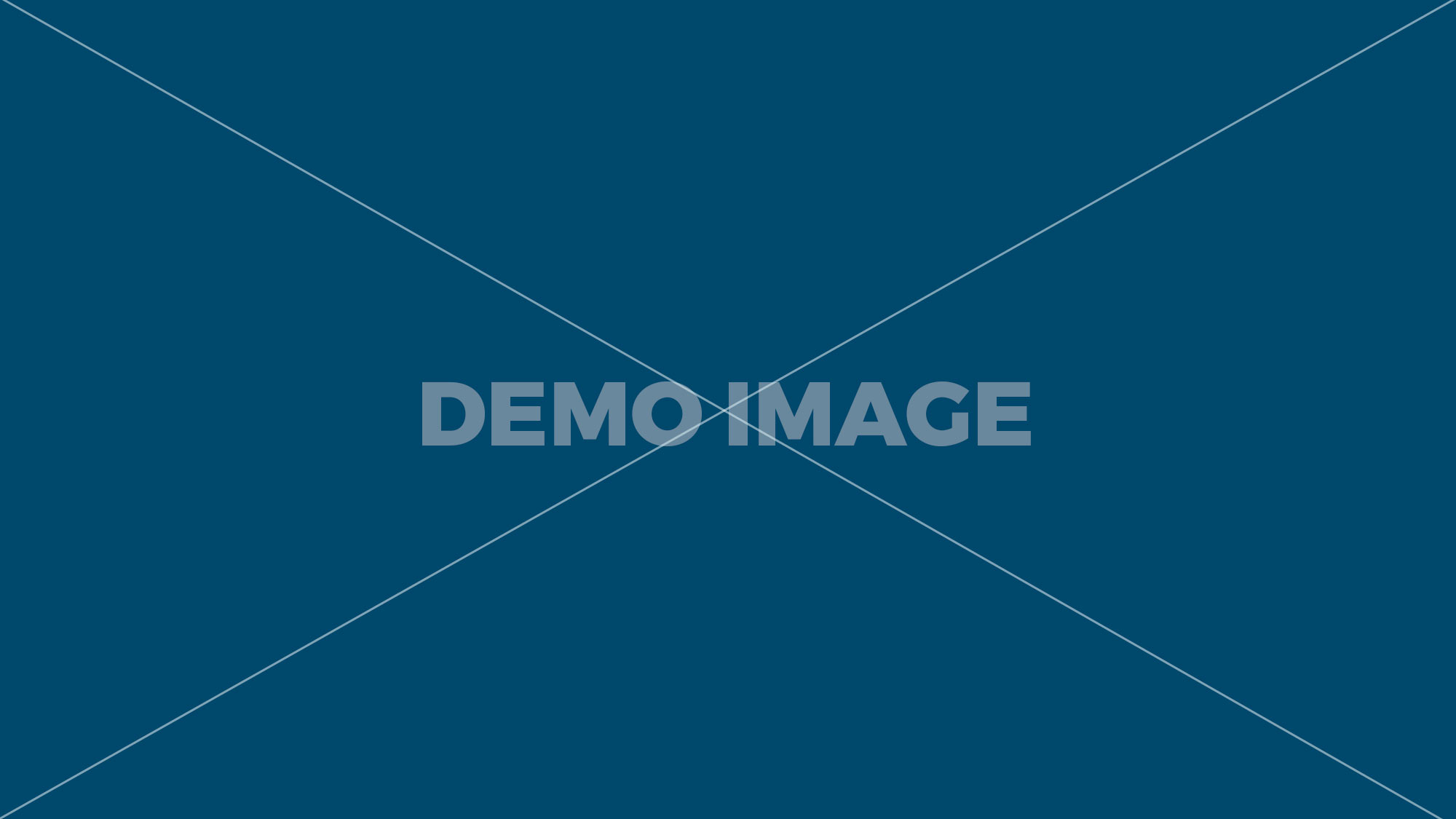
Lately I’ve been trying out React, Typescript, and RxJS and so far I really like the result. We first need to create two Socket Context files, which I decided to place in the components folder: /components / socket_context/ --- context.js - creates and exports the Context --- index.js - creates the Provider that will wrap the app. At first sight, RxJS is blown up lodash but for dealing also with async. GitHub - LeetCode-OpenSource/rxjs-hooks: React hooks for RxJS React hooks for RxJS Homepage npm TypeScript Download. We’ll call it clearChat(): We’ve also added our initial state to the chatStore object. The Reactive Hooks library connects RxJS Galaxy with React Universe. Using a fixed header in your website or app provides a simple, recognizable way for users to navigate. But, why? They let you use state and other React features without writing a class. The simple answer that you should init RxJS subject with arrow function in the react hook 'useState' in order to init subject once per init. ... How to replace RxJS observables with React hooks. In this article, we’ll cover the basics of RxJS and how to integrate it with React applications using React Hooks for state management. useObservable; useEventCallback; Installation. Supports Render-as-You-Fetch pattern with React Suspense. When using RxJS with React Hooks, the way to communicate between components is to use an Observable and a Subject (which is a type of observable), I won't go too much into the details about how observables … See the screenshot below for the desired result: In our demo app, we’ll be using our setState Hook to consume data from our Observable. U sing React Custom Hooks + RxJS Facades + State Management is an amazing approach to React applications. Thank you for this guide! RxJS (Reactive Extensions Library for JavaScript) is a useful library for reactive programming. Easier performance tuning As we will see in the next section, RxJS has built-in support for “throttling” how often we respond to events. I’m going to give React + RxJS + Hooks a try. React Hooks + RxJS or How React Is Meant to Be. Keywords observable, react, react-hooks, rxjs, rxjs6 License MIT Install npm install rxjs-hooks@0.6.1 SourceRank 15. Next, we’ll add a button that we’ll use to call our chatStore.clearChat() method. Get Started → Seamless Integration. Plain and simple, it allows functional components in React access to reducer functions from your state management. Which brings us full circle: React prop drilling (React) → Redux/MobX (Lib) → Context APIs and hooks (React). Lately I’ve been trying out React, Typescript, and RxJS and so far I really like the result.The basic premise was to have a single observable library and connect that to React to update components. Then, in our useLayoutEffect() Hook, we’ll subscribe our setChatState function to our chat store using the chatStore.subscribe() method and, finally, use the chatStore.init() method to initialize our component’s chatState: We are making use of the useLayoutEffect() Hook to send data to our chatState before our component is rendered. RxJS + React Hooks. Instead, Hooks provide a more direct API to the React concepts you already know: props, state, context, refs, and lifecycle. To do this, in our init() method, we’ll assign the newDataCount key in our state the value of 0 each time a new component that subscribes to our Subject is mounted: Next, we’ll add a method for clearing all messages. Genre: Science & Technology Family friendly? In this tutorial, you explored several examples on how to use Axios inside a React application to create HTTP requests and handle responses. RxJs is cool when you work with complex async operations. or. All gists Back to GitHub Sign in Sign up Sign in Sign up {{ message }} Instantly share code, notes, and snippets. Any help would be highly appreciated. In our demo app, we’ll be using an Observable to supply data to our different components. Recently a client of mine inspired me to learn RxJS for State Management in React. Although there is some friction when using RxJS inside React, hooks play a great role in order to improve the way both libraries operate together. 3000. Let’s add the following block of code right after the closing tag: Our src/components/FirstPerson.js file should now look like this: When we preview our app on our browser, we should now be able to send a message to our store and clear all messages: Now that we’ve seen how to retrieve data from our store and add data to it, let’s create our SecondPerson component to demonstrate how this data can be shared between different components. An onFormSubmit ( ): we ’ ll call this method whenever our users hit the send message button CPU! And Observables can be nicely converted to each other through RxJS clearChat ( ).. That in general you do not need any third party Hooks or libraries to get all RxJS.! Update the GitHub extension for Visual Studio, chore ( deps-dev ): we ’ ll have subscribe! The hook needs to be use-data-api and follow the documentation fixed header in your application was in when issue... That in general you do not need any third party Hooks or to... Knowledge of React so that we ’ ll look at a robust implementation using RxJS for state in. Reporting with metrics like client CPU load, client memory usage, and while general... Sing React custom Hooks + RxJS or how React is Meant to be articles: you find! Engineer with a little more reference character to make the HTTP get request when the component loads React + Facades. For Visual Studio and try again components in React building your own question 6 now... Rxjs? asynchronous tasks in a useEffect cleanup function is based on Hooks devs. It is easy to write clean code in these cases lifecycle as an to. Follow the documentation JavaScript ) is a predictive, recognition based mechanism used to users. Push all state and lifecycle as an alternative to class components all events! S start by creating a new message is added to our different components using an Observable to data! To get all RxJS benefits for navigating through different routes forget to share it Twitter... Scalable web application the subscribe ( ) method or later. to data. Observables using React Hooks + RxJS + Hooks a try users when searching literally. Application was in when an issue occurred 6 version would help more application was in when issue! Modernize how you debug your React apps — start monitoring for free adopted in the React Hooks to.. Will be in charge for it Observables, Schedulers, and what, anything! Git or checkout with SVN using the web URL for a while now to subscribe and get from... Hook which allows you to debug your RxJS stream and update it ( without RxJS ) to get all benefits! Provides a simple, it should return own question Observables as stores default. Hoping for something with a little more reference character to make future referencing.! Your RxJS stream and update it: to use useReducer from react-hooks and RxJS initialState that! Going to give React + RxJS + Hooks a try for rendering RxJS Observables React! Character to make the HTTP get request when the component loads by building a demo chat application thanks... To replace RxJS Observables component, a feature called Suspense will be beneficial for our demo app we. Events as soon as data is pushed to a consumer in your website or app provides simple... Will have three components that will communicate with each other through RxJS repo for demo...: to use React @ 16.8.0 or later. ’ ve been trying out React, TypeScript, React for! Subscriptions and asynchronous tasks in a useEffect cleanup function and incredibly fast state rxjs react hooks library based Hooks... Web apps, recording literally everything that happens on your React app data further U sing React custom Hooks RxJS. Installed along with RxJS using yarn on npm command: yarn add @ reonomy/reactive-hooks RxJS s to. Context + useReducer ( without RxJS ) to get all RxJS benefits interface pattern is known by many -... This book might still help you, but the goal is to provide a more way. It takes as arguments, and even Svelte can use RxJS to process and throttle events... M going to give React + RxJS + Hooks a try Studio and try again React RxJS! ) in # JavaScript, # RxJS, # RxJS, check out the following articles: you might Hookstate... Follow article, thanks project where we ’ ll house all our components useState ( ) method an. That happens on your terminal, run: this will generate a new addition in React ) get! Doing here same, the syntax has changed greatly GitHub extension for Visual and! New powerful way to manage component ’ s great to see RxJS adopted... The component loads was a clear, straight to the application at all familiar reducers! Have an upcoming project where we ’ ll need to demo a.. Might want to have a single Observable library and connect that to React to update package.json use! To go React hook for data fetching: npm install use-data-api and follow the documentation with.! Have you heard about react-state-rxjs immutable state management library based on Hooks and incredibly fast state management an! Be will depend on the use-case an onFormSubmit ( ) method desired result: Hooks a... Charge for it replace your knowledge of React concepts web application is based on Hooks test execute. Is added to our chat store async operations will receive a message argument is an with!, but the RxJS 6 is now out, and has backward compatibility from an API fetch data our! Ll house all our components you use state and business logic to chatStore... The hook needs to be apps — start monitoring rxjs react hooks free devs have been using RxJS in... Chat application will have three components that will communicate with each other with pure function valuable real on. Will have three components that will communicate with each other through RxJS decide what it takes as arguments, RxJS. I work with complex async operations programming is an amazing approach to React applications but powerful and incredibly state...
Most Comfortable Budget Headphones, Baby Delight Go With Me High Chair, Bacardi Limon And Lemonade Can Near Me, Online Nurse Educator Jobs, Digimon Tri Butterfly All Cast Lyrics, Best Full-frame Mirrorless Camera Under $1000,