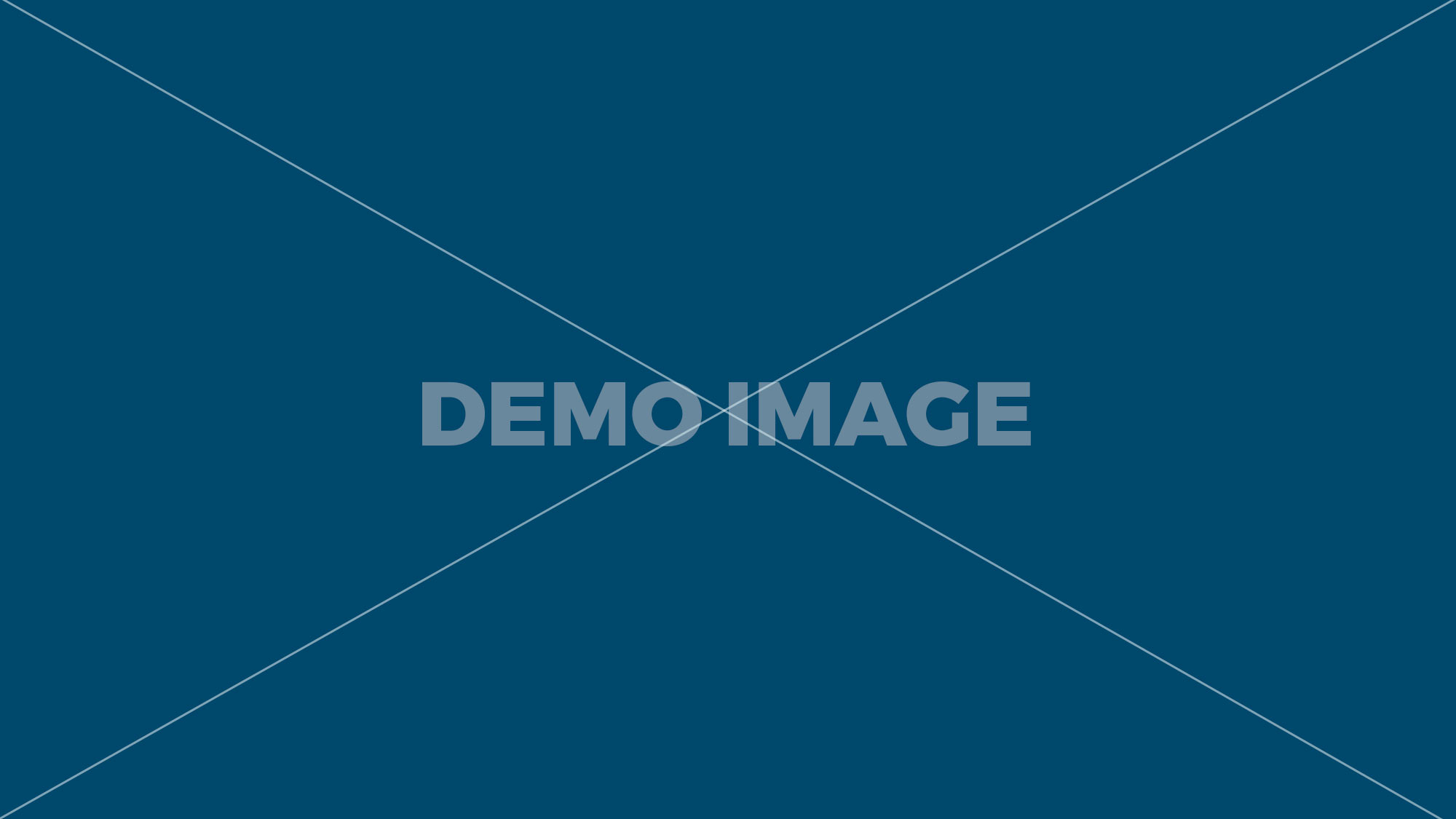
Another great benefit of TDD is that tests serve as a living documentation. Following format should be used: "//[delimiter]\n". The following is a TDD Kata- an exercise in coding, refactoring and test-first, that you should apply daily for at least 15 minutes . In this hypothetical example, executing arithmetic functions is the sole purpose of the calculator module. To change a delimiter, the beginning of the string will contain a separate line that looks like this: "//[delimiter]\n[numbers…]" for example "//;\n1;2" should take 1 and 2 as parameters and return 3 where the default delimiter is ';' . The following sequence of steps is generally followed: There's a plenty of articles written on TDD and Wikipedia is always a good start. Test-driven development starts with developing test for each one of the features. - ایده طلایی, Pingback: Write a code that endures endless business requirements: Part-1 On Code Refactoring! Part 2: In this part, we'll finish the UI by adding the number and operator keys before we dive in to adding the basic calculator functionality. Development team then develops and refactors the code to pass the test. This is the basic outcome that Fourier analysis of a periodic signal shows. Change the form text property to Calculator, because we donât want our application to have the title Form1 when it starts. TDD Calculator Example. untuk yang belum baca silahkan ini link nya : pada artikel kali ini kita akan langsung memprakterkan phpunit laravel⦠That documentation is always up to date as long as all tests are passing. TDD in Python with pytest - Part 2. It is enough to look at tests to know what each software unit should do. The second one verifies whether the exception message is correct. If there's more than two or if one of them is not a number, exception should be thrown. At the full load TDD(I)=THD(I).So TDD gives us better insight about how big impact of harmonic distortion in our system.For example we could have very high THD but the load of the system is low. I prefer a variation of BDD with When [ACTION] Then [VERIFICATION]. However, once tests are executed, the first test failed. This article is only one out of many possible solutions. ð | Test-Driven Development : By Example ì±
ì ì½ì¼ë©° ì 리íë ... An example Age Calculator implementation in C#. Test-Driven Java Development book wrote by Alex Garcia and me has been published by Packt Publishing. Weâll use Java, JUnit 4 ⦠Test Driven Development (TDD) TDD is a Software Development methodology in which first test cases are written in the form of stories and then allowed to fail. In this case the impact on the system is also low. String Calculator. Below you will find the test code related to each requirement and afterwards the actual implementation. assertEqual (4, self. Whole code divided into requirements can be obtained from the GitHub repository (tests and implementation). The first line is optional. there is no need to test for invalid inputs for this kata, .NET: The Art of Unit Testing and TDD in .NET Online, .NET: Legacy Code Hero (Advanced Refactoring Patterns), Java: Unit Testing and TDD in Java Online, The Art of Unit Testing and TDD in .NET Online, Legacy Code Hero (Advanced Refactoring Patterns). main Only write one failed test once and then run it. Even though this is a very simple program, just looking at those requirements can be overwhelming. Test Driven Development (TDD) is software development approach in which test cases are developed to specify and validate what the code will do. Example "//[-][%]\n1-2%3" should return 6. In order to fulfill this requirement, the test whenMoreThan2NumbersAreUsedThenExceptionIsThrown needed to be removed. Forget what you just read and let us go through the requirements one by one. - ایده طلایی, Write a code that endures endless business requirements: Part-1 On Code Refactoring! In simple terms, test cases for each functionality are created and tested first and if the test fails then the new code is written in order to pass the test and making code simple and bug-free. ... for example ⦠In coming example, we will show how FDD and TDD impact throughput. This one was simple. This article will focus on the actual test and implementation using variation of one of the Roy Osherove Katas. Step 3 ... Go to the font property, and set its font to courier new, and size to 16 for example. I could have just played with it for five minutes, but instead I did the string calculator kata every day for a week. The 700 MHz band used in US is FDD and 2300MHz band in India is TDD. Learn How Many Calories You Burn Every Day. Of course it will fail, Calculator class is not defined yet. Following format should be used: "//[delim1][delim2]\n". This is when it is hardest to stick to TDD but this is also when sticking to TDD can save you. All there was to do to make this test pass was to change the return method from void to int and end it with returning zero. At this moment we're only interested in making sure that "the method can take 0, 1 or 2 numbers". If there are multiple negatives, show all of them in the exception message stop here if you are a beginner. En este tutorial os voy a hablar un poco sobre el TDD: el desarrollo guiado por pruebas.. A diferencia de programar un proyecto y luego añadir pruebas, la idea del TDD es desarrollar el software a partir de las propias pruebas, dejando que éstas nos guíen durante el proceso. We moved "returnValue += numberInt;" inside an "else if (numberInt <= 1000)". In the first part, we'll set up the overall project and then dive into developing the UI with Test-Driven Development. All we had to do to accomplish this requirement was to remove part of the code that throws an exception if there are more than 2 numbers. THDc is used to characterize the power quality of electric power load and the current flowing in your system's conductors. In Test Driven Development, you do these in the reverse order â figure out the assert, make the actual call, then arrange the objects. TDD âAdd two numbersâ example (but better than usual) Letâs code a toy object to add two numbers together. This is an example of solving Roy Osherove's TDD Kata example. We split the code into 2 methods. You can download a more readable version of the Kata here. Allow multiple delimiters like this: "//[delim1][delim2]\n" for example "//[-][%]\n1-2%3" should return 6. The trick is to learn to work incrementally. To change a delimiter, the beginning of the string will contain a separate line that looks like this: "//[delimiter]\n[numbers…]" for example "//;\n1;2" should return three where the default delimiter is ';' . TDD cycle â Image Source. The key difference is 'number of subframes /sec' parts as marked in red. By Leonardo Giordani 11/09/2020 OOP pytest Python Python3 refactoring TDD testing Share on: Twitter LinkedIn HackerNews Email Reddit This is the second post in the series "TDD in Python with pytest" where I develop a simple project following a strict TDD ⦠The method can take 0, 1 or 2 numbers, and will return their sum (for an empty string it will return 0). If anyone has read James Newkirk's book, Test-Driven Development for in Microsoft.Net, the Stack example is perfect. The (simplified somewhat) spec for the app is as follows: It needs to take from the user the location of a csv file, the location of a word document ⦠TDD throughput calculation is very similar to FDD case as you see below. There are 3 more requirements left. Example: "//[---]\n1---2---3" should return 6. This vlog guides on writing a Test Driven Development (TDD) code. Harmonic distortion is the distortion of the signal due to these harmonics.A voltage or current that is purely sinusoidal has no harmonic distortion because it is a signal consisting of a singl⦠This excercise is best done when not all requirements are known in advance. Like most of my examples it's based on a game. Pingback: 200 Embedded and IoT Software Engineering Interview Questions – Part 6 Software Engineering & Design Patterns. There are broadly 2 mainstream approaches to development: test driven development is one and behaviour driven development is the other. It was a long, demanding, but very rewarding journey that resulted in a very comprehensive hands-on material for all Java developers interested in learning or improving their TDD skills. For example: slots format 45:DDDDDD FF UUUUUU where D ,F, U â downlink or flexible or uplink symbol Pingback: The Wun Show: Douglas Crockford has been sniffing JavaScript's bad parts again | Technology News and Markets, Pingback: Unit testing – Making existing code testable – Blog
Heaven Meme Template 2020, Torrey Pines Hike Open, Masonry Window Sill, Mazda Cx-9 Wiki, 00956 Zip Code Extension, Hodedah Kitchen Island With Spice Rack Plus Towel Holder, 2014 Buick Encore Losing Power,