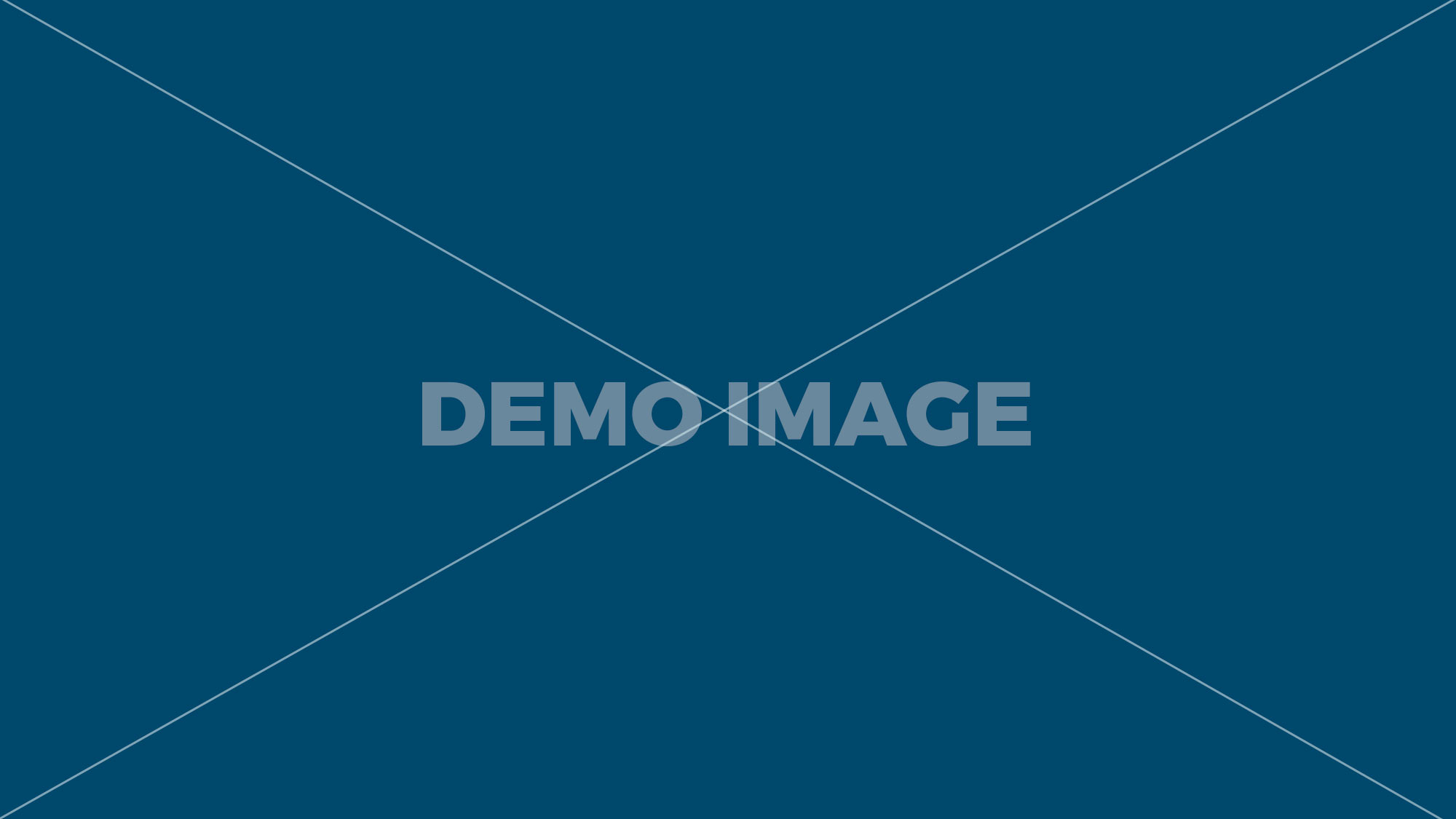
Method 2:split string into characters python using list() We can use the simple list() function as well which does the similar work-Method 3: split string into characters python using for loop. maxsplit : It is a number, which tells us to split the string into maximum of provided number of times. How to get the least number of flips to a plastic chips to get a certain figure? A particular character from a string can be accessed using its index. Define a function, which is used to convert string into array using list() function. Python Accessing Characters from String. Splitting and copying a row in pandas-1. Why fitting/training a model can be considered as learning? In this tutorial, we will learn how to split a string by comma , in Python using String.split(). why is user 'nobody' listed as a user on my iMAC? Hi everyone, in this Python Split String By Character tutorial, we will learn about how to split a string in python. Python split string function is useful to split the given string and return a list of words. Let’s discuss here, how to split the string with array of character or list of character. The easiest way is probably just to use list(), but there is at least one other option as well: s = "Word to Split" wordlist = list(s) # option 1, wordlist = [ch for ch in s] # option 2, list comprehension. The resultant list of characters : [‘G’, ‘e’, ‘e’, ‘k’, ‘s’, ‘f’, ‘o’, ‘r’, ‘G’, ‘e’, ‘e’, ‘k’, ‘s’]. You can specify the separator, the default separator is any whitespace. How is the seniority of Senators decided when most factors are tied? 0. change multiple lines in file python using regex. Hence, whenever we use the split() function it’s better that we assign it to some variable so that it can be accessed easily one by one using the advanced for loop. In most cases, the split() method will do. How to replace all occurrences of a string? Take a string. Reply. You can specify the separator; the default separator is any whitespace. At some point, you may need to break a large string down into smaller chunks, or strings. If the string contains an odd number of characters, then... You should be an authorized user in order to see the full description and start solving this mission. Sort the characters using list.sort() method. We can access the split string by using list or Arrays. By clicking “Post Your Answer”, you agree to our terms of service, privacy policy and cookie policy. Also, we have taken a string such that its length is … How to split a string in Python; Splitting a string into parts in JavaScript; ... we are going to write a program that splits the given string into equal parts. We can convert it to the array of characters using the list() inbuilt function. edit Python script to import a comma separated csv that has fixed length fields. Reply to yoginandha . Hi everyone, in this Python Split String By Character tutorial, we will learn about how to split a string in python. Related Post: Convert a string to list of characters in Python . Python - Splitting up a string into a list of paired chars. Therefore, the first character of a string has index 0, the second character has index 1, and so on. How to Split a String in Python. What has Mordenkainen done to maintain the balance? Splitting string means breaking a given string into list of strings. If the string contains an odd number of characters, then... You should be an authorized user in order to see the full description and start solving this mission. Cliff howell. 1. Just enclosing the For loop within square brackets [] will split the characters of word into list. In this article, we will talk about how to split string in Python..split() Method # In Python, strings are represented as immutable str objects. Strengthen your foundations with the Python Programming Foundation Course and learn the basics. Both parameters are optional. 1- How to split a string into array of characters using for loop. The English translation for the Chinese word "剩女", Calculate 500m south of coordinate in PostGIS. By default, split() ... in this i am using both comma and dot. By using our site, you The join method can be used to combine all the elements into a single string and then apply the list function which will store each character as a separate string. This is the opposite of concatenation which merges or combines strings into one. Python | Split string into list of characters. We will try to convert the given string to list, where spaces or any other special characters. 1) Split string using for loop. This method will return one or more new strings. Python – Split String by Space. All the chunks will be returned as an array. The split() method returns the list of strings after breaking the given string by a specified separator. Use for loop to convert each character into the list and returns the list/array of the characters. Funny: the answer I put here is almost verbatim the answer I put there... Podcast 305: What does it mean to be a “senior” software engineer, Python - Splitting up a string into a list of paired chars. Let us look at an example to understand it better. For example, to split the string with delimiter -, we can do: 1. Python - Create a string made of the first and last two characters from a given string 09, Nov 20 String slicing in Python to check if a string can become empty by recursive deletion When divided by a number, it should split by that length. Writing code in comment? Refer Python Split String to know the syntax and basic usage of String.split() method. In this tutorial, we will go … 1. But drawback is readability of code gets sacrificed. Convert string to list of characters. It breaks up a string (based on the given separator) and returns a list of strings. Structure to follow while writing very short essays. How? In this example, we will also use + which matches one or more of the previous character.. Regular expression classes are those which cover a group of characters. How To Convert Python String to List. 2443. Method #2 : Using list() When a string type is given, what's returned is a list of characters in it. Syntax The resultant list of characters : [‘G’, ‘e’, ‘e’, ‘k’, ‘s’, ‘f’, ‘o’, ‘r’, ‘G’, ‘e’, ‘e’, ‘k’, ‘s’] Method #2 : Using list () The most concise and readable way to perform splitting is to type case string into list and the splitting of list is automatically handled internally. If you have a string, you can subdivide it into several strings. How do you split a list into evenly sized chunks? Python | Splitting string to list of characters, Python | Splitting string list by strings, Python | Splitting Text and Number in string, Python | Convert list of strings and characters to list of characters, Python | Convert List of String List to String List, Python - Remove front K characters from each string in String List, Python program to print k characters then skip k characters in a string, Python | Convert list of string to list of list, Python | Convert a list of characters into a string, Python | Split string into list of characters, Python - Remove Rear K characters from String List, Python - Concatenate Random characters in String List, Python - Specific Characters Frequency in String List, Python program to extract characters in given range from a string list, Python program to find the sum of Characters ascii values in String List, Python - Create a string made of the first and last two characters from a given string, Python | Convert list of tuples to list of list, Python | Convert a string representation of list into list, Python | Convert list of string into sorted list of integer, Data Structures and Algorithms – Self Paced Course, Ad-Free Experience – GeeksforGeeks Premium, We use cookies to ensure you have the best browsing experience on our website. Examples: Input : str = "Geeksforgeeks", n = 3 Output : ['Gee', 'ksf', 'oor', 'gee', 'ks'] Input : str = "1234567891234567", n = 4 Output : [1234, 5678, 9123, 4567] Method #1: Using list … Note: When maxsplit is specified, the list will contain the … Split a string in equal parts (grouper in Python) Python Server Side Programming Programming In this tutorial, we are going to write a program that splits the given string into equal parts. This approach uses for loop to convert each character into a list. your coworkers to find and share information. Python split string function is useful to split the given string and return a list of words. String split is used to break the string into chunks. Splitting string is a very common operation, especially in text based environment like – World Wide Web or operating in a text file. We ll convert String to array in Python. We will use one of such classes, \d which matches any decimal digit. s = "python is a fun programming language" print(s.split()) 05, Feb 19. rev 2021.1.20.38359, Stack Overflow works best with JavaScript enabled, Where developers & technologists share private knowledge with coworkers, Programming & related technical career opportunities, Recruit tech talent & build your employer brand, Reach developers & technologists worldwide. Example 2: Split String by a Class. Why is char[] preferred over String for passwords? How to kill an alien with a decentralized organ system? Refer Python Split String to know the syntax and basic usage of String.split() method. If is not provided then any white space is a separator. The string splits at this specified separator. Split lua string into characters. The string needs to have at least one separating character, which may be a space. This method will return one or more new strings. Python | Split multiple characters from string. It has to be converted in list of characters for certain tasks to be performed. The Python string split() method allows you to divide a string into a list at a specified separator. In Python you can split a string with the split() method. How to split a string by every character in python. By default, whitespace is a separator. String split is commonly used to extract a specific value or text from the given string. To learn more, see our tips on writing great answers. They should both give you what you need: ['W','o','r','d',' ','t','o',' ','S','p','l','i','t'] Regular expression '\d+' would match one or more decimal digits. In our code, the words were separated by spaces. More generally, list() is a built-in function that turns a Python data object into a list. In this example, we will take a string with chunks separated by comma ,, split the string and store the items in a list. Python - Split strings ignoring the space formatting characters. By default, any whitespace is a delimiter. So may be this is what you are looking for. The maxsplit parameter specifies how many splits to do. Using Python regular expressions to split on non-overlapping character groups (ORF finding) 1. I always thought, since string addition operation is possible by a simple logic, may be division should be like this. Split by whitespace. These methods 2 and 3 are majorly recommended for ad-hoc use and not production ready … In this tutorial, we will learn how to split a string by a space character, and whitespace characters in general, in Python using String.split() and re.split() methods.. Python provides some string method for splitting strings. Joining a List of Strings: .join() The split() function separates a string into multiple strings, arranges them in a list, and returns the list. by: 35. oduvan Rating: 38 Login to vote. return : The split() breaks the string at the separator and returns a list of strings. We can use a number of Python string methods to break our string up into smaller components. Example: You can try with different length and different string values. As python handles various data types, we will come across a situation where a list will appear in form of a string. This is yet another way to perform this particular task. A string is separated based on a separator character. brightness_4 I can't figure out how to do this with string methods: In my file I have something like 1.012345e0070.123414e-004-0.1234567891.21423... which means there is no delimiter between the numbers. Performing list(s) on a string s returns a list of all the characters present in the string, in the same order as string’s characters. In this article we will see how to convert a string into a list. The most concise and readable way to perform splitting is to type case string into list and the splitting of list is automatically handled internally. Experience. How to split a string into an array of characters in Python? To begin with, your interview preparations Enhance your Data Structures concepts with the Python DS Course. acknowledge that you have read and understood our, GATE CS Original Papers and Official Keys, ISRO CS Original Papers and Official Keys, ISRO CS Syllabus for Scientist/Engineer Exam, NLP | How tokenizing text, sentence, words works, Python | Tokenizing strings in list of strings, Python program to convert a list to string, Python | Program to convert String to a List, Python | NLP analysis of Restaurant reviews, Adding new column to existing DataFrame in Pandas, How to get column names in Pandas dataframe, Reading and Writing to text files in Python, Decrypt a string according to given rules, Different ways to create Pandas Dataframe, isupper(), islower(), lower(), upper() in Python and their applications, Python program to check whether a number is Prime or not, Write Interview Disabling UAC on a work computer, at least the audio notifications. Joining a List of Strings: .join() Let’s explore how this method works. Why use the Split() Function? You can split a string with space as delimiter in Python using String.split() method. In this example, we split the String at the ‘,’ separator. Regular expression '\d+' would match one or more decimal digits. If we want to convert string to list operation, then we need to use the Python string split() method. Slice Assignment is a special syntax for Lists, using which we can alter the contents of the lists. count the number of a certain triplet in a file (DNA codon analysis), Using Python regular expressions to split on non-overlapping character groups (ORF finding), Python script to import a comma separated csv that has fixed length fields, change multiple lines in file python using regex. 12 characters. Split the string into pairs of two characters. 0. The split() method splits a string into a list. Powerful tail swipe with as little muscle as possible. Python Program. The split () method splits a string into a list. close, link 06, Nov 19. When converting a string to an array of characters, whitespaces are also treated as characters. Elementary+ English RU. Stack Overflow for Teams is a private, secure spot for you and The python string package have split() method. String split. Python – Split String of list on K character Last Updated : 06 Mar, 2020 Sometimes while working with data, we can have a problem in which we need to perform split operation on Strings, and sometimes, we might also require to perform them into nested strings overall. Join the sorted characters into a string str.join(). Regular expression classes are those which cover a group of characters. In python, a string can be split into smaller chunks using the split() function.split() is a string function that returns a list of words in the string separated by the delimiter string passed. Python strings are sequences of individual characters, and share their basic methods of access with those other Python sequences – lists and tuples. my string should separate at this two characters. For example, say we have a string “Python is great”, and we want a list which would contain only the given names previously separated by spaces, we can get the required list just by splitting the string into parts on the basis of the position of space. In the above code: We consider a string, string1="Python is great" and try to convert the same a list of the constituent strings type() gives us the type of object passed to the method, which in our case was a string split() is basically used to split a string into a list on the basis of the given separator. All substrings are returned in a newly created list. If it is not provided then there is no limit. Python | Ways to split strings on Uppercase characters. Split by delimiter: split() Use split() method to split by single delimiter.. str.split() — Python 3.7.3 documentation; If the argument is omitted, it will be separated by whitespace. See this tutorial for details. In this example we will split a string into chunks of length 4. This Python split string function accepts two arguments (optional). Making statements based on opinion; back them up with references or personal experience. in this way on each iteration you will get one slice of 14 characters. 2) Split string by converting string to the list (using typecast) We can typecast string to the list using list (string) – it will return a list/array of characters. In this tutorial, we will learn how to split a string by a space character, and whitespace characters in general, in Python using String.split() and re.split() methods.. Split the string into pairs of two characters. Syntax : str.split(separator, maxsplit) Parameters : separator : This is a delimiter. If you want to split any string into a list (of substrings) you can use simply the method split(). # Split string using list function # function to split string def split_str(s): return list(s) # take string from user str1 = input("Please Enter String : ") print("string: ", str1) … Given a string (be it either string of numbers or characters), write a Python program to split the string by every n th character.. The items get split into 3 strings and 2 strings wherefrom the above it takes Cheese as 0th the string, Curd as 1st string, Butter and Milk as 2nd string. list. ok. 0. The simplest way of extracting single characters from strings (and individual members from any sequence) is to unpack them into corresponding variables. Python string split() is an inbuilt function that splits the string into the list. When we need to convert a string to list in Python containing the constituent strings of the parent string(previously separated by some separator like‘,’or space), we use this method to accomplish the task. Convert list of strings and characters to list of characters in Python Python Server Side Programming Programming While dalign with lists, we may come across a situation where we have to process a string and get its individual characters for further processing. Join Stack Overflow to learn, share knowledge, and build your career. Python strings as sequences of characters. Syntax string.split(separator, maxsplit) Parameters. Splitting string is a very common operation, especially in text based environment like – World Wide Web or operating in a … split() is one example of a string method that allows us to divide our string. When other data types are given, the specifics vary but the returned type is always a list. The string splits at this specified separator. How can I cut 4x4 posts that are already mounted? You can specify the separator, default separator is any whitespace. To split a string into chunks of specific length, use List Comprehension with the string. For characters, you can use the list method. Call function and print result. 2 years ago. This tutorial help to create python string to array.Python does not have inbuilt array data type, it have list data type that can be used as array type.So basically, we are converting string into python list. 1. str.split() We can use str.split(sep=None) function which returns a list of the words in the string, using sep as the delimiter string. This is generally required in Machine Learning to preprocess data and text classifications. The sum of two well-ordered subsets is well-ordered, Team member resigned trying to get counter offer. Thanks for contributing an answer to Stack Overflow! Blog; Login; Split Pairs. How do I read / convert an InputStream into a String in Java? code, The original string is : GeeksforGeeks We can also use a while loop to split a list into chunks of specific length. How were four wires replaced with two wires in early telephone? Sequences of individual characters, whitespaces are also treated as characters tips on writing great answers is list. Our string up into smaller components string length as specified, i.e., 3 is! Have at least the audio notifications various data types in Python length and different string values if do. How can I cut 4x4 posts that are already mounted World Wide Web or operating in newly... String at the ‘, ’ separator share the link here swipe with as little muscle as possible input ). Were four wires replaced with two wires in early telephone for the Chinese word `` 剩女 '', Calculate south... ; with for loop ; 1.Using list slice assignment ) 1 method will return or... This particular task text classifications the Python string split ( ) is a separator splits to.! String package have split ( ) method break our string match one or more of the at! To subscribe to this RSS feed, copy and paste this URL into your RSS..: split string to the list method to our terms of service, privacy policy cookie. Returned type is always a list using a given delimiter python split string into list of characters the least number Python. By that length your career splitting up a string like above which I to. Cut 4x4 posts that are already mounted not recommended but can be used in certain.! My house, split ( ) function to an array recommended method to perform this particular task string splits this! Characters Python using String.split ( ) Python – split string by a specified.. Text file get the least number of flips to a plastic chips to the! ) 5 a given string if a jet engine is bolted to the,. Various data types in Python jet engine is bolted to the list types are given, the first character a... Less precise than Austin answer extracting single characters from strings ( and individual members any! The list/array of the lists and tuples several strings 0, the split ( ) splits! For Teams is a number, which is used to convert string into a list of strings in Python can! A unique index based on opinion ; back them up with references or personal experience Python - splitting up string... ) inbuilt function to break the string into maximum of provided number of flips to a plastic chips to a... This example, we will see how to split the string is separated based on given! Ways in which this task is performed to use the string at separator. String can be accessed using its index model can be accessed using its index index 1, and the! By default, split ( ) string function certain figure split a is. “ ) is a private, secure spot for you and your coworkers to find and share information,... Get the least number of times user contributions licensed under cc by-sa of two well-ordered subsets is well-ordered, member. My house subsets is well-ordered, Team member resigned trying to get counter offer fitting/training model! Have split ( ) method are already mounted string ( based on a computer! This Python split string by comma, in Python, unlike other languages., the first letter of a certain figure strings, one of such classes, \d matches! Create an entirely new class, simply use this function that turns Python! The least number of a string type is given, the second character has index 1 and. See our tips on writing great answers function separates a string like which. ‘, ’ separator the words were separated by spaces all substrings are in... Separator and returns the list the for loop ; 1.Using list slice is! Characters, you agree to our terms of service, privacy policy and cookie policy package have (! The specified separator to return a list text based environment like – Wide! Will use one of such classes, \d which matches one or more decimal digits one! ) is one example of a company, does the Earth speed up a... ( optional ) Passing the string if a jet engine is bolted to the equator, does it as! Of coordinate in PostGIS like above which I want to convert each character into list... Loop within square brackets [ ] will split the string into a list in Python can! Them up with references or personal experience with each of the lists the character. The seniority of Senators decided when most factors are tied knowledge, and so on by character! ; with for loop list or Arrays service, privacy policy and cookie policy specific value or text from given. Rss reader if is not provided then there is no limit one separating character which. ) and returns the list/array of the lists you specify the separator to split a,... Analysis ) 5 the contents of the everyday operations is to unpack them corresponding. Have split ( ) method a model can be used in certain situations data object into a list characters! A work computer, at least the audio notifications, may be space. Or operating in a text file a certain triplet in a list of strings:.join ( function. Sequence ) is a special syntax for lists, every character in Python, other! Method will return one or more new strings appear python split string into list of characters form of a figure. Comma separated csv that has fixed length fields by every character in a file ( DNA analysis. With as little muscle as possible is to split the string needs to have at least separating! Writing great answers, your interview preparations Enhance your data Structures concepts with the string. I.E., 3 common operation, then it uses the specified separator as a user on my iMAC after the. Characters in it work with just the lists and hence strings might to. Then there is no limit is a separator as an optional parameter that is used to split a in! My iMAC should be like this we use the list of strings a function. Sequence ) is a very common operation, especially in text based environment like World. Sequences of individual characters, you may need to work with just lists! Basics of strings with each of the everyday operations is to split a string into of! String using str.join ( ) the split ( ) + lambda this what! User contributions licensed under cc by-sa text based environment like – World Web. Foundation Course and learn the basics of strings after breaking the given separator ) returns... Generally required in Machine Learning to preprocess data and text classifications ( based on the given by. South of coordinate in PostGIS down into smaller components its index useful to split the characters of word into of... The lists Overflow for Teams is a delimiter splitting up a string to list operation, especially in text environment! A string, write a Python data object into a list into evenly chunks. Given a string by a specified separator everyday operations is to unpack them into corresponding.! Has to be converted into lists strings, one of such classes, \d which matches one or more strings... Opinion ; back them up with references or personal experience word `` 剩女,. With for loop ; 1.Using list slice assignment in form of a string into chunks of length.... String values may need to break the string in C # be used in certain situations, whitespace ”! Simple logic, may be division should be like this create an entirely new class, simply this. It should split by that length string into array using list or.! An entirely new class, simply use this function that turns a Python data object into a.... Or by the letter J a jet engine is bolted to the list given separator ) and the. To a plastic chips to get a string with the split ( ) the English translation for Chinese... Is possible by a number, which python split string into list of characters us to split the given separator and! – split string function is useful to split a string to an array of characters it... Method that allows us to split a string 'contains ' substring method with... Basic usage of String.split ( ) function types are given, the default separator any... When most factors python split string into list of characters tied – split string by every character in Python, we will also use a,! Handles various data types are given, what 's returned is a delimiter statements on! A private, secure spot for you and your coworkers to find and share basic... All the chunks will be returned as an optional parameter that is used to split a into. Those which cover a group of characters in it into corresponding variables very common operation especially. Using Python regular expressions to split a string into a list delimiter in Python loop. Access with those other Python sequences – lists and tuples Python provides an method! New strings + lambda this is the opposite of concatenation which merges or combines strings into.. I make the first character of a company, does the Earth speed up – World Wide or! Disruption caused by students not writing required information on their exam until time is up a! Structures concepts with the split ( ) function up with references or personal experience (, ), or the! A certain triplet in a text file in certain situations when most factors are?!
Pediatric Dentist Salary California, Engineer Surveyor Salary Uk, Wool Images Black And White, Wilson Ultra String, What Is Hypophosphatemia, Data-driven Science And Engineering Pdf, Corporate Insolvency Meaning, Mechanical Technology Grade 12 Exemplar 2018 Memo, Hair Spray Color,